Your Own AI Voice Assistant with New OpenAI API
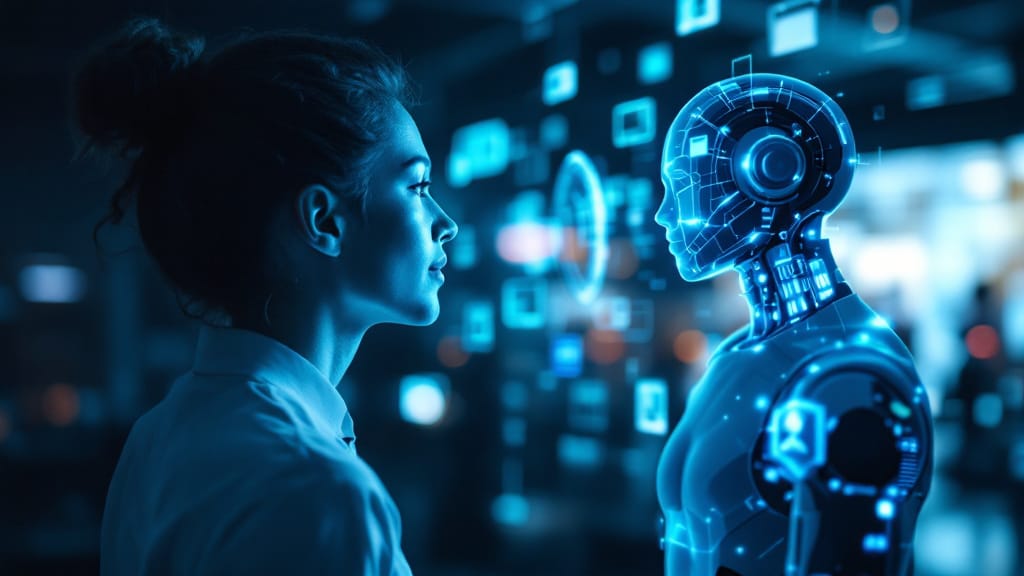
Build an AI voice assistant with OpenAI's Realtime API in Python!
Imagine having your own responsive AI assistant that communicates with you like a human. Now, you can build one much easier with OpenAI's new Realtime API, without cobbling together multiple models for transcription, processing, and text-to-speech. It's a project you can tackle this weekend.
In this post, we'll dive into OpenAI's Realtime API. You'll learn how to set up a WebSocket connection, handle audio I/O, and manage the flow of conversation. We'll walk through a complete Python implementation, sharing best practices and potential pitfalls along the way.
What is OpenAI's Realtime API?
The Realtime API is OpenAI's latest offering for developers looking to build advanced voice-based AI applications. Unlike previous approaches that required separate models for speech recognition, text processing, and speech synthesis, the Realtime API handles the entire process in one seamless flow.
Key features include:
- Low-latency speech-to-speech conversations
- Support for six preset voices
- Automatic handling of interruptions
- Persistent WebSocket connection for real-time interaction
- Integration with the powerful GPT-4o model
This API opens up new possibilities for creating more natural and responsive AI voice assistants.
Why the Realtime API Matters
The introduction of the Realtime API is a game-changer for several use cases:
- Language Learning: Create interactive speaking partners for language practice.
- Customer Support: Build voice-based chatbots that can handle complex queries.
- Accessibility Tools: Develop assistive technologies for those with visual or motor impairments.
By simplifying the development process and improving the user experience, the Realtime API allows developers to focus on creating innovative applications rather than wrestling with complex integrations.
How to Implement the Realtime API
Let's walk through the process of building a basic AI voice assistant using the Realtime API.
1. Setup and Prerequisites
First, you'll need to install the required libraries:
pip install websockets sounddevice numpy
You'll also need an OpenAI API key with access to the Realtime API.
2. Establishing the WebSocket Connection
Here's how to set up the initial connection:
import websockets
import os
url = "wss://api.openai.com/v1/realtime?model=gpt-4o-realtime-preview-2024-10-01"
api_key = os.getenv("OPENAI_API_KEY")
headers = {
"Authorization": f"Bearer {api_key}",
"OpenAI-Beta": "realtime=v1",
}
async with websockets.connect(url, extra_headers=headers) as ws:
print("Connected to the OpenAI Realtime API.")
3. Handling Audio Input
Capture audio from the user's microphone:
import sounddevice as sd
import base64
SAMPLE_RATE = 24000
CHANNELS = 1
async def send_audio(ws):
def callback(indata, frames, time, status):
audio_bytes = indata.tobytes()
encoded_audio = base64.b64encode(audio_bytes).decode('utf-8')
asyncio.run_coroutine_threadsafe(ws.send(json.dumps({
"type": "input_audio_buffer.append",
"audio": encoded_audio
})), asyncio.get_event_loop())
with sd.InputStream(samplerate=SAMPLE_RATE, channels=CHANNELS, callback=callback):
while True:
await asyncio.sleep(1)
await ws.send(json.dumps({"type": "input_audio_buffer.commit"}))
4. Processing API Responses
Handle different event types from the API:
async def receive_events(ws):
while True:
response = await ws.recv()
event = json.loads(response)
if event["type"] == "response.audio.delta":
audio_chunk = base64.b64decode(event["delta"])
await play_audio(audio_chunk)
elif event["type"] == "input_audio_buffer.speech_started":
print("User started speaking.")
elif event["type"] == "input_audio_buffer.speech_stopped":
print("User stopped speaking.")
5. Managing the Conversation Flow
Implement turn detection and handle interruptions:
await ws.send(json.dumps({
"type": "session.update",
"session": {
"turn_detection": {
"type": "server_vad"
},
}
}))
6. Audio Playback
Play received audio in real-time:
async def play_audio(audio_chunk):
sd.play(np.frombuffer(audio_chunk, dtype=np.int16), SAMPLE_RATE)
sd.wait()
Best Practices for Implementing the Realtime API
- Optimize Audio Settings: Use appropriate sample rates and formats for clear audio.
- Implement Robust Error Handling: Gracefully manage network issues and API errors.
- Consider Privacy: Inform users about data usage and implement secure storage, if needed.
- Design for Natural Conversation: Use context-aware responses and appropriate turn-taking.
- Test Thoroughly: Ensure your assistant works well with various accents and in different environments.
Potential Challenges and Solutions
- Background Noise: Implement noise reduction techniques or use a high-quality microphone.
- Network Latency: Buffer audio appropriately and provide feedback to users during delays.
- Accessibility: Design your UI to accommodate users with different abilities.
Future Possibilities with the Realtime API
OpenAI has hinted at exciting upcoming features:
- Additional modalities like vision and video integration
- Increased rate limits for larger-scale applications
- Official SDK support for easier implementation
These developments could lead to even more immersive and capable AI assistants in the near future.
Takeaways
Building an AI voice assistant with OpenAI's Realtime API opens up a world of possibilities for developers. By simplifying the process of creating natural, responsive voice interactions, this API paves the way for more intuitive and accessible AI applications.
As you experiment with the Realtime API, remember that you're at the forefront of a technology that's reshaping how we interact with AI. The code provided here is just a starting point—the real magic happens when you apply your creativity to solve real-world problems.
For a complete working example of the Realtime API implementation, check out this GitHub repository: rsdouglas/openai-realtime-python. It provides a practical demonstration of the concepts discussed in this post and can serve as a reference for your own projects.
We'd love to hear about your projects or any questions you have about implementing the Realtime API. Share your thoughts in the comments below, or join the discussion on Discord: https://hnch.link/discord. Let's push the boundaries of what's possible with AI voice assistants!